Next.js: Behaviour of getInitialProps with SSR
Following the tutorials, getInitialProps
is meant to work “on both server and the client”, which is totally correct.
Using server-side rendering (SSR) without any enhancements, this leads to a pwa, which is pre-baked for seo crawlers (content within html markup), but still loads content by the api, even if all data is within the rendered page already.
So be aware of implementing some client/server logic, when loading content by api is needed, and when it can be avoided.
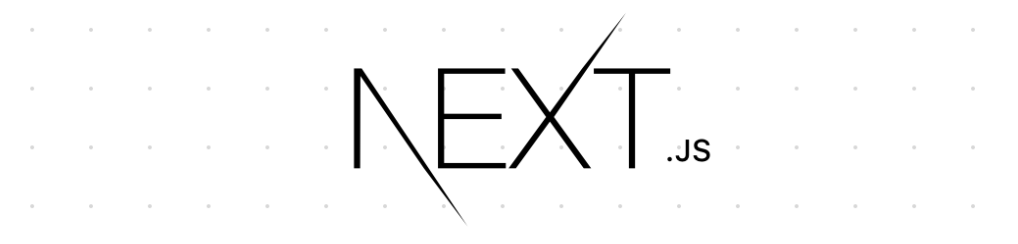
What we will need, is to force loading data from api while ssr, avoid api requests if data is already pre-baked and still load content from api in browser, if no ssr did the job before:
Page.getInitialProps = async function(context) {
const { id } = context.query;
if (!context.req) {
console.log('if running in browser, check if props are already loaded by ssd');
if(window.__NEXT_DATA__.props.pageProps.show){
console.log('browser > NEXT_DATA exists');
const show = window.__NEXT_DATA__.props.pageProps.show
console.log(show);
if(show.id == id){
console.log('browser > props are there');
return { show }
}
}
console.log('browser > props could not be found');
}
console.log('getting props from api, independent from server or browser runtime');
const res = await fetch(`https://api.tvmaze.com/shows/${id}`);
const show = await res.json();
return { show };
};
This code should just illustrate the requirement and the basic concept, which is needed to provide best performance while staying robust on missing or long-tail-content ssr routes.